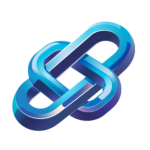
NLTK (Natural Language Toolkit) - Detailed Review
Analytics Tools
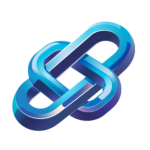
NLTK (Natural Language Toolkit) - Product Overview
The Natural Language Toolkit (NLTK)
NLTK is a versatile and widely-used Python library dedicated to natural language processing (NLP). Here’s a brief overview of its primary function, target audience, and key features:
Primary Function
NLTK is designed to facilitate the development of applications for statistical natural language processing. It enables computers to manipulate and comprehend human language, performing tasks such as text processing, tokenization, parsing, classification, and semantic reasoning.
Target Audience
NLTK is suitable for a broad range of users, including translators, educators, researchers, and those in industrial applications. It is particularly useful for computational linguistics classes, helping students and professionals alike to develop and work with NLP components and systems.
Key Features
Tokenization and Text Preprocessing
NLTK allows you to break down text into smaller units such as words or sentences. It also provides tools for removing stop words, stemming, and lemmatization, which are essential steps in text analysis.
Part-of-Speech Tagging and Named Entity Recognition
NLTK includes tools for identifying the parts of speech in sentences (e.g., nouns, verbs, adjectives) and recognizing named entities like people, organizations, and locations.
Sentiment Analysis
The toolkit can determine the sentiment of a given piece of text, which is useful for applications like social media monitoring or product review analysis.
Support for Multiple Languages
NLTK is not limited to English; it supports a wide range of languages, including Arabic, Chinese, French, German, Hindi, Italian, Japanese, and more.
Integration with Other Libraries
NLTK can be used in conjunction with other machine learning libraries such as scikit-learn and TensorFlow, allowing for more sophisticated NLP applications.
Educational Resources
NLTK comes with a curriculum, a book, and various tutorials, making it an excellent tool for teaching and learning computational linguistics.
Overall, NLTK provides a comprehensive framework for NLP tasks, making it an invaluable resource for both beginners and advanced users in the field.
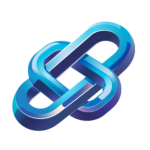
NLTK (Natural Language Toolkit) - User Interface and Experience
The Natural Language Toolkit (NLTK)
The Natural Language Toolkit (NLTK) is a comprehensive library for natural language processing (NLP) in Python, but it does not provide a traditional user interface in the way many other analytics tools do. Here’s how it works and what the user experience is like:
Command-Line and Script-Based Interface
NLTK is primarily used through Python scripts and the command line. Users interact with NLTK by writing Python code to import the library, load corpora, and perform various NLP tasks such as tokenization, part-of-speech tagging, parsing, and sentiment analysis.
Ease of Use
While NLTK is highly powerful, its ease of use can vary depending on the user’s familiarity with Python and NLP concepts. For those new to NLP, NLTK provides extensive documentation, including a book titled “Natural Language Processing with Python” and numerous online resources and tutorials. These resources help guide users through the fundamentals of writing Python programs for NLP tasks.
User Experience
The user experience with NLTK is centered around coding and scripting. Here are some key aspects:
- Interactive Shell: Users can use the Python interactive shell to experiment with NLTK functions and see immediate results. This interactive environment is helpful for testing and debugging code.
- Extensive Documentation: NLTK comes with comprehensive API documentation, HOWTO guides, and examples that make it easier for users to learn and use the library effectively.
- Community Support: NLTK has an active community, which means there are many resources available online, including forums, tutorials, and blogs, to help users overcome any challenges they might encounter.
- Corpora and Data: NLTK provides access to over 50 corpora and lexical resources, which are essential for training and testing NLP models. This extensive collection of data helps users in their NLP projects.
Engagement and Factual Accuracy
For users engaged in NLP tasks, NLTK offers a practical and hands-on approach. The library’s focus on providing clear, step-by-step examples and its interactive nature help ensure factual accuracy in the results obtained from NLP analyses.
Conclusion
In summary, while NLTK does not have a graphical user interface, it is designed to be highly accessible and usable through Python scripting, making it a valuable tool for anyone working with natural language data.
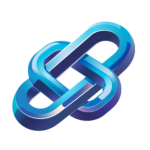
NLTK (Natural Language Toolkit) - Key Features and Functionality
The Natural Language Toolkit (NLTK)
The Natural Language Toolkit (NLTK) is a comprehensive and widely-used library in Python for natural language processing (NLP). Here are the main features and functionalities of NLTK, along with explanations of how each works and their benefits:
Tokenization
Tokenization is the process of breaking down text into smaller units called tokens, which can be words, sentences, or even characters. NLTK provides functions like word_tokenize
and sent_tokenize
to perform word and sentence tokenization, respectively. This step is crucial for most NLP tasks as it prepares the text data for further processing.
Part-of-Speech (POS) Tagging
POS tagging involves assigning grammatical tags to words in a sentence, such as nouns, verbs, and adjectives. NLTK offers pre-trained models for POS tagging, which can be used to analyze the grammatical structure of sentences. Here is an example of how to use POS tagging with NLTK:
import nltk
sentence = "Welcome to Educative!"
words = nltk.word_tokenize(sentence)
part_of_speech_tags = nltk.pos_tag(words)
print(part_of_speech_tags)
Named Entity Recognition (NER)
NER identifies and classifies named entities in text into predefined categories such as names, organizations, and locations. NLTK provides tools for NER, which can be integrated with other NLP tasks. Here’s an example of performing NER:
import nltk
from nltk import word_tokenize, pos_tag, ne_chunk
text = "Barack Obama was born in Hawaii."
words = word_tokenize(text)
pos_tags = pos_tag(words)
named_entities = ne_chunk(pos_tags)
print(named_entities)
Stemming and Lemmatization
These techniques reduce words to their base or root forms. Stemming uses algorithms to cut off the ends of words, while lemmatization uses a dictionary to find the base form of a word. NLTK supports both stemming and lemmatization through libraries like PorterStemmer
and WordNetLemmatizer
:
from nltk.stem import PorterStemmer
stemmer = PorterStemmer()
input_word = "processing"
stemmed_word = stemmer.stem(input_word)
print(stemmed_word)
from nltk.stem import WordNetLemmatizer
lemmatizer = WordNetLemmatizer()
print("rocks :", lemmatizer.lemmatize("rocks")) # Output: "rock"
WordNet
WordNet is a lexical database included in NLTK that provides a semantic network of words and their relationships. It can be used to find synonyms, antonyms, and other related words.
from nltk.corpus import wordnet
synonyms_output = []
for s in wordnet.synsets("good"):
for lemma in s.lemmas():
synonyms_output.append(lemma.name())
print(synonyms_output)
Sentiment Analysis
NLTK facilitates sentiment analysis, which determines the sentiment or opinion expressed in text. This can be particularly useful for analyzing user opinions and emotions in social media data or customer feedback.
from nltk.sentiment import SentimentIntensityAnalyzer
sia = SentimentIntensityAnalyzer()
sentence = "Welcome to Educative!"
sentiment_scores = sia.polarity_scores(sentence)
print(sentiment_scores)
Text Classification
NLTK offers tools for text classification, enabling the building of models that can categorize text into predefined classes. This is useful for tasks like spam detection or sentiment analysis.
from nltk.classify import NaiveBayesClassifier
from nltk.tokenize import word_tokenize
# Sample data
sentences = []
Parsing and Syntax Analysis
NLTK provides functions for parsing sentences to understand their grammatical structure, which is essential for tasks like part-of-speech tagging and dependency parsing.
from nltk.corpus import treebank
t = treebank.parsed_sents('wsj_0001.mrg')
t.draw()
Information Extraction
NLTK enables the extraction of structured information from unstructured text, aiding in tasks like named entity extraction and relation extraction. This is crucial for applications that need to extract specific data from large volumes of text.
Machine Translation
While NLTK itself does not perform machine translation, it can be integrated with other libraries to prepare text data for translation tasks.
Text Summarization
NLTK can summarize large amounts of text by extracting critical information and producing concise summaries. This is useful for tasks that require condensing lengthy documents into key points.
Integration with NLP Models
NLTK can be integrated with various NLP models, including machine learning and deep learning frameworks like TensorFlow or PyTorch. After tokenization and filtering, the processed tokens can be fed into these models for further analysis.
from sklearn.feature_extraction.text import CountVectorizer
# Example tokenized sentences
corpus = []
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(corpus)
X_array = X.toarray()
print(X_array)
Applications
- Chatbots and Virtual Assistants: NLTK powers the NLP capabilities of chatbots and virtual assistants, enabling them to comprehend and respond to user queries.
- Social Media Sentiment Analysis: NLTK can analyze sentiment in social media data, helping understand user opinions and emotions.
- Language Learning and Teaching: NLTK can be used for language learning and teaching purposes, assisting in vocabulary acquisition, grammar analysis, and exercises.
In summary, NLTK is a versatile and powerful tool for NLP tasks, offering a wide range of functionalities that can be integrated into various AI-driven applications to enhance text processing and analysis capabilities.
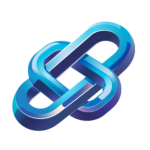
NLTK (Natural Language Toolkit) - Performance and Accuracy
Performance and Accuracy of NLTK in AI-Driven Analytics
When evaluating the performance and accuracy of NLTK (Natural Language Toolkit) in the context of AI-driven analytics tools, several key points need to be considered.Sentiment Analysis
NLTK offers multiple approaches for sentiment analysis, including rule-based and machine learning methods. The Vader sentiment analysis tool, which is part of NLTK, is a popular rule-based approach that provides a pre-trained model for analyzing sentiment. This tool can achieve reasonable accuracy, with an example showing an overall accuracy of 79% in a specific use case. However, the accuracy can vary depending on the dataset and the specific requirements of the project. For instance, machine learning-based approaches, which require labeled training data, can potentially offer better accuracy but are more computationally expensive and require more resources.Named Entity Recognition (NER)
For NER tasks, NLTK is useful but has its limitations. It is particularly suited for teaching purposes and small-scale projects due to its ease of use and the availability of pre-trained models. However, NLTK may not perform as well as deep learning approaches like RNNs, LSTMs, or transformers (e.g., BERT), which can achieve higher accuracy and efficiency in identifying entities.Text Preprocessing
NLTK is effective for various text preprocessing tasks such as tokenization, stop word removal, stemming, and lemmatization. However, it can be slower compared to newer libraries like spaCy, especially when dealing with very large datasets or real-time text analysis. Effective preprocessing with NLTK can significantly enhance the performance and accuracy of machine learning models by providing cleaner, more relevant data.Limitations
Performance
NLTK may not be ideal for processing very large datasets or for real-time text analysis due to its slower performance compared to other libraries.Scalability
NLTK’s performance and scalability issues make it less suitable for large-scale or complex NLP tasks, where deep learning methods might be more appropriate.Language Support
Tokenization and other NLP tasks in NLTK can be challenging for languages that do not use white spaces or punctuation to separate words, such as Chinese, Japanese, or Arabic.Areas for Improvement
Integration with Deep Learning
While NLTK can be used as a preliminary step for preprocessing data, integrating it with deep learning frameworks like TensorFlow or PyTorch can enhance its capabilities. However, this integration may require additional steps to convert the processed data into suitable formats.Community Updates
NLTK benefits from regular updates from its community of contributors, which can introduce new features, improved algorithms, and bug fixes. This ongoing development helps in enhancing its functionality and efficiency.Conclusion
In summary, NLTK is a powerful and flexible library for various NLP tasks, including sentiment analysis and NER, but it has limitations in terms of performance, scalability, and support for certain languages. For more complex or large-scale projects, combining NLTK with deep learning approaches or using other specialized libraries might be necessary to achieve optimal results.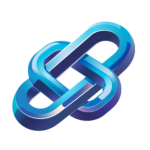
NLTK (Natural Language Toolkit) - Pricing and Plans
Overview of The Natural Language Toolkit (NLTK)
The Natural Language Toolkit (NLTK) is a free, open-source library for Natural Language Processing (NLP) in Python, and it does not have a pricing structure or different tiers of plans. Here are the key points:
Free and Open-Source
NLTK is completely free to use, making it accessible to anyone interested in NLP without any financial barriers.
No Tiers or Plans
There are no different tiers or plans for NLTK. It is a single, comprehensive package that includes a wide range of tools and resources for text processing, tokenization, part-of-speech tagging, named entity recognition, and more.
Extensive Features
NLTK provides a broad set of features, including interfaces to corpora like WordNet, text processing libraries for classification, tokenization, stemming, tagging, parsing, and semantic reasoning. It also includes wrappers for industrial-strength NLP libraries and an active community-driven development.
Community and Documentation
NLTK is supported by extensive documentation, including a book, API documentation, and various HOWTOs with examples and test cases. This makes it suitable for a wide range of users, from students and educators to researchers and industry professionals.
Conclusion
In summary, NLTK is a free resource with no pricing structure or different plans, offering a rich set of NLP tools and resources to its users.
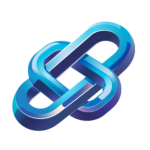
NLTK (Natural Language Toolkit) - Integration and Compatibility
The Natural Language Toolkit (NLTK)
The Natural Language Toolkit (NLTK) is a versatile and widely-used library for natural language processing (NLP) that integrates well with various tools and maintains a high level of compatibility across different platforms and devices.Integration with Other Libraries
NLTK can seamlessly integrate with other popular NLP libraries. For instance, it can be used in conjunction with libraries like SpaCy and Gensim, which is beneficial for developers working on complex NLP problems. This integration allows for a comprehensive approach to NLP tasks, leveraging the strengths of each library. For example, NLTK’s extensive text corpora and lexical resources can be combined with SpaCy’s high-performance capabilities in tokenization and entity recognition. Additionally, NLTK functions can be accessed through simpler interfaces provided by libraries like TextBlob, which is an extension of NLTK. TextBlob simplifies many of NLTK’s functions, making it easier for beginners to use while still being suitable for production environments for smaller projects.Compatibility with Programming Languages
NLTK is primarily written in Python, a popular language for NLP applications. This makes it highly compatible with other Python-based tools and libraries. However, NLTK can also interface with other programming languages such as Java and C, providing flexibility for developers working in multi-language environments.Platform Compatibility
NLTK can be installed and run on various platforms that support Python. The installation process is straightforward using the Python package installer, `pip`. You can install NLTK by running `pip install nltk` in your command prompt or terminal. After installation, you can download the necessary text corpora and resources using the `nltk.download()` function.Handling Dependencies
One of the considerations when using NLTK is managing its dependencies, particularly the text corpora and lexical resources. While `pip` can install the NLTK library itself, it cannot download these additional resources. To handle this, you can use the `nltk.download()` function to download the specific datasets you need. For deployment in certain environments, such as Streamlit or Heroku, listing the required NLTK dependencies in a `nltk.txt` file can be a useful workaround.Educational and Real-World Applications
NLTK’s compatibility and integration capabilities make it a valuable tool in both educational settings and real-world applications. It is widely used in academic research, sentiment analysis, chatbot development, and language education. Its ability to integrate with other libraries and tools ensures that it remains a cornerstone in the field of NLP, even as newer libraries like SpaCy gain popularity.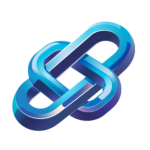
NLTK (Natural Language Toolkit) - Customer Support and Resources
Customer Support Options for NLTK
When considering the customer support options and additional resources provided by NLTK (Natural Language Toolkit), it’s important to note that NLTK is primarily a library and toolkit for natural language processing, rather than a customer support platform. Here are some key points regarding what NLTK offers:Resources and Corpora
NLTK provides access to over 50 corpora and lexical resources, such as WordNet, Penn Treebank, and TIMIT Corpus, among others. These resources are invaluable for researchers, students, and developers working with human language data.Documentation and Guides
NLTK offers comprehensive documentation, including a book that introduces programming fundamentals alongside topics in computational linguistics. This documentation is suitable for linguists, engineers, students, educators, and industry users. The guides cover various aspects of text processing, such as tokenization, stemming, tagging, parsing, and semantic reasoning.Community Support
NLTK has an active discussion forum where users can seek help, share knowledge, and collaborate on projects. This community support is crucial for resolving issues and learning from others who are using the toolkit.Data Access and Download
Users can download various corpora and data packages using NLTK’s data downloader. This includes the ability to download individual data packages or the entire collection, which can be useful for teaching and research purposes.Tools and Libraries
NLTK includes a suite of text processing libraries that can be used for classification, tokenization, stemming, tagging, parsing, and semantic reasoning. These tools are essential for building Python programs that work with human language data. While NLTK does not provide traditional customer support options like live chat or phone support, it offers extensive resources and a supportive community that can help users overcome challenges and make the most out of the toolkit.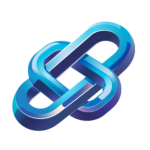
NLTK (Natural Language Toolkit) - Pros and Cons
Advantages of NLTK
NLTK, or the Natural Language Toolkit, is a highly regarded and widely used library in the Python NLP community, offering several significant advantages:
Comprehensive Features
NLTK provides a wide range of NLP tasks, including tokenization, part-of-speech (POS) tagging, named entity recognition (NER), sentiment analysis, and access to various corpora.
Extensive Corpora
NLTK has an extensive collection of corpora, which includes text data from diverse sources such as books, news articles, and social media platforms. This rich data source is invaluable for training and testing NLP models.
Educational and Research Use
NLTK is particularly popular in education and research due to its comprehensive nature and the ease with which it can be used for various NLP tasks. It has led to many breakthroughs in text analysis.
Support for Multiple Languages
NLTK supports the largest number of languages compared to other NLP libraries, making it versatile for multilingual text analysis.
Ease of Use for Specific Tasks
NLTK offers easy-to-use interfaces for specific combinations of algorithms, making it an excellent choice when you need to perform particular NLP tasks.
Disadvantages of NLTK
Despite its many advantages, NLTK also has some notable disadvantages:
Steep Learning Curve
NLTK can be difficult to learn and use, especially for beginners. The library requires a good understanding of its various components and how to integrate them.
Performance Issues
NLTK is known to be slow, which makes it less suitable for large-scale production usage. This is a significant drawback when speed and efficiency are critical.
Lack of Neural Network Models
NLTK does not include neural network models, which can limit its accuracy and capabilities compared to more modern NLP libraries that leverage deep learning techniques.
Limited Semantic Analysis
NLTK primarily splits text by sentences without deeply analyzing the semantic structure, which can be a limitation in certain applications.
In summary, while NLTK is a powerful and comprehensive tool for NLP tasks, its slow performance, lack of neural network models, and steep learning curve are important considerations when deciding whether to use it for your specific needs.
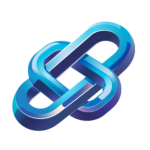
NLTK (Natural Language Toolkit) - Comparison with Competitors
When Comparing NLTK with Other Tools
When comparing NLTK (Natural Language Toolkit) with other tools in the AI-driven analytics category, several key points and alternatives come to the forefront.
Unique Features of NLTK
- Comprehensive Toolset: NLTK offers a broad range of NLP tools, including tokenization, stop words removal, stemming, lemmatization, part-of-speech tagging, named entity recognition, and parsing. This makes it highly versatile for various NLP tasks such as sentiment analysis, text classification, and information extraction.
- Educational Focus: NLTK is highly regarded for its educational value, providing extensive documentation and a hands-on guide that introduces programming fundamentals alongside computational linguistics. This makes it an excellent choice for students, researchers, and educators.
- Large Corpora and Resources: NLTK includes access to over 50 corpora and lexical resources like WordNet, which is beneficial for training and testing NLP models.
- Integration with Machine Learning: NLTK integrates seamlessly with machine learning libraries, allowing for the development and testing of classifiers for various NLP tasks.
Comparison with SpaCy
- Performance: SpaCy is known for its speed and efficiency, processing text up to 20 times faster than NLTK. This makes SpaCy ideal for large-scale text processing and production-grade data pipelines.
- Ease of Use: SpaCy has a more user-friendly API and is easier to integrate with other tools like pandas and numpy. NLTK, while powerful, may require more effort for beginners to get started.
- Pre-trained Models: SpaCy comes with pre-trained models that are highly accurate and efficient, whereas NLTK requires more manual setup and customization.
Potential Alternatives
- SpaCy: As mentioned, SpaCy is a strong alternative for those needing high-performance NLP processing. It is particularly useful for tasks that require speed and integration with other data science tools.
- Gensim: Gensim is another library that focuses on topic modeling and document similarity analysis. It is efficient for handling large volumes of text data and can be used in conjunction with NLTK for more comprehensive NLP tasks.
Use Cases
- Research and Education: NLTK is widely used in research and educational settings due to its extensive resources, flexibility, and educational focus. It allows for more experimentation and customization, making it ideal for those exploring different NLP techniques.
- Production Environments: For production environments where speed and efficiency are critical, SpaCy might be a better choice. However, NLTK can still be used in conjunction with SpaCy to leverage its broader range of NLP tools and resources.
In summary, NLTK stands out for its comprehensive toolset, educational value, and extensive resources, making it a great choice for research, education, and exploratory NLP tasks. However, for high-performance and production-grade NLP, alternatives like SpaCy may be more suitable.
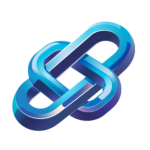
NLTK (Natural Language Toolkit) - Frequently Asked Questions
Here are some frequently asked questions about NLTK, along with detailed responses to each:
Q: What is NLTK and what is it used for?
NLTK, or the Natural Language Toolkit, is a Python library used for statistical natural language processing (NLP). It provides tools and resources for tasks such as tokenization, parsing, classification, stemming, and semantic reasoning, making it a powerful tool for creating NLP applications.
Q: How do I install NLTK and its data?
To install NLTK, you can use Python’s package manager. After installing NLTK, you need to download the necessary data using NLTK’s data downloader. You can do this by running `import nltk` and then `nltk.download()` in a Python interpreter. You can also specify the download location and use command-line or manual installation methods if needed.
Q: What are the key features of NLTK for text preprocessing?
NLTK offers several key features for text preprocessing, including tokenization, stemming, lemmatization, removing stop words, and part-of-speech tagging. These features help in cleaning and structuring text data, which is essential for effective NLP tasks.
Q: Can NLTK be used for sentiment analysis?
Yes, NLTK includes tools for sentiment analysis. It allows you to determine the sentiment of a given piece of text, which is useful for applications such as social media monitoring or product review analysis.
Q: How does NLTK handle different languages?
NLTK supports a wide range of languages, not just English. It provides tools for tokenization, stemming, and morphological analysis for languages such as Arabic, Chinese, Dutch, French, German, Hindi, Italian, Japanese, Portuguese, Russian, Spanish, and more.
Q: Can NLTK be integrated with other machine learning libraries?
Yes, NLTK can be used in conjunction with other machine learning libraries such as scikit-learn and TensorFlow. This integration allows for more sophisticated NLP applications, including deep learning-based language modeling.
Q: What are the limitations of using NLTK for text preprocessing?
NLTK can be slower compared to newer libraries like spaCy, and it may not be ideal for processing very large datasets or for real-time text analysis. However, it remains a valuable tool for many NLP tasks and is particularly useful for educational purposes and smaller-scale projects.
Q: How important is text preprocessing in NLP tasks?
Text preprocessing is crucial for NLP tasks as it standardizes text data, reduces complexity, and improves the accuracy and reliability of analysis. The necessity of each preprocessing step depends on the specific NLP task at hand.
Q: Can NLTK preprocessing tools be automated?
Yes, you can create scripts and functions in Python using NLTK to automate various text preprocessing tasks. However, the extent of automation might depend on the complexity and variability of the text data.
Q: Are there any specific hardware requirements for running NLTK?
NLTK is not particularly resource-intensive and can run on standard hardware configurations. However, the overall performance might depend on the complexity and volume of the text data being processed.
Q: How often is NLTK updated, and how does it impact its functionality?
NLTK is an open-source project and receives regular updates from its community of contributors. These updates can introduce new features, improved algorithms, and bug fixes, enhancing its overall functionality and efficiency.
Q: Can NLTK be used in web applications?
Yes, NLTK can be used in the backend of web applications for text preprocessing tasks. It can be integrated into web application frameworks like Django or Flask to process text data received from web users.
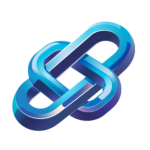
NLTK (Natural Language Toolkit) - Conclusion and Recommendation
Final Assessment of NLTK in the Analytics Tools AI-Driven Product Category
The Natural Language Toolkit (NLTK) is a highly versatile and powerful tool in the domain of natural language processing (NLP). Here’s a comprehensive assessment of NLTK, including its benefits and the types of users who would benefit most from using it.Key Features and Capabilities
Text Processing
NLTK offers a wide range of text processing tools, including tokenization, stemming, part-of-speech tagging, parsing, and semantic reasoning. It supports multiple languages, making it a global tool for NLP tasks.
Corpora and Resources
NLTK provides easy-to-use interfaces to over 50 corpora and lexical resources like WordNet. This extensive library is invaluable for training and testing NLP models.
Sentiment Analysis and Classification
NLTK includes tools for sentiment analysis, text classification, and named entity recognition, which are crucial for applications such as social media monitoring, product review analysis, and customer feedback analysis.
Integration with Other Libraries
NLTK can be used in conjunction with other machine learning libraries like sci-kit-learn and TensorFlow, enabling more sophisticated NLP applications, including deep learning-based language modeling.
Who Would Benefit Most
Researchers and Academics
NLTK is an excellent tool for educational purposes, providing a hands-on guide to programming fundamentals and computational linguistics. It is widely used in teaching and research environments.
Developers and Engineers
Developers working on NLP projects can leverage NLTK’s extensive libraries and resources to build applications such as chatbots, sentiment analyzers, and text classifiers.
Business Analysts and Marketers
For those involved in market analysis, customer feedback, and social media monitoring, NLTK’s capabilities in sentiment analysis and text classification can provide valuable insights into customer sentiments and preferences.
Overall Recommendation
NLTK is a must-have tool for anyone working in the field of NLP. Here are some key reasons why:
Community Support
NLTK has a large and active community of users and contributors, ensuring a wealth of resources, including online forums, tutorials, and example codes, are available for learning and troubleshooting.
Ease of Use
Despite its advanced capabilities, NLTK is relatively easy to get started with, especially for those familiar with Python. It provides comprehensive documentation and a practical guide to NLP tasks.
Cost-Effective
As an open-source project, NLTK is free to use, making it an accessible option for both individuals and organizations.
In summary, NLTK is an indispensable tool for anyone involved in natural language processing. Its extensive features, ease of use, and strong community support make it an excellent choice for a wide range of NLP applications. Whether you are a researcher, developer, or business analyst, NLTK can help you extract valuable insights from text data and build sophisticated NLP models.