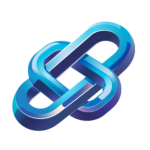
Gradio - Detailed Review
Developer Tools
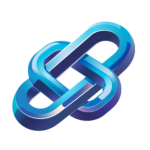
Gradio - Product Overview
Introduction to Gradio
Gradio is an open-source Python library that simplifies the creation of interactive web interfaces for machine learning models, APIs, and any Python functions. Here’s a brief overview of its primary function, target audience, and key features:Primary Function
Gradio’s main purpose is to enable developers to build user-friendly web interfaces for their machine learning models, APIs, or any Python functions. This allows end-users, who may not be technically inclined, to interact with these models, provide input, and observe results in real-time.Target Audience
Gradio is particularly useful for machine learning engineers, data scientists, and Python developers who need to demonstrate their models to a broader audience. It is also beneficial for educators and anyone looking to make complex machine learning models accessible to non-technical users.Key Features
Interactive Components
Gradio offers a suite of interactive components such as Textbox, Buttons, and Sliders, which facilitate the creation of intuitive and responsive user interfaces. These components allow users to input data, trigger specific actions, and customize the interface as needed.Easy Setup and Deployment
Gradio makes it easy to build and deploy web applications with minimal code. It supports server-side rendering, which ensures applications load quickly in the browser, and it includes built-in themes for a fresh and modern look.Integration and Automation
Gradio integrates well with other Python tools and frameworks, such as Hugging Face’s Transformers library. The Gradio API allows for remote interactions, automation, and testing, making it versatile for various use cases, including deploying machine learning models as remote services.Performance and Security
Gradio 5 introduces several enhancements, including performance improvements through server-side rendering, enhanced security measures validated by Trail of Bits, and AI-powered app creation through the AI Playground. It also supports low-latency streaming, which is useful for real-time applications like webcam-based object detection and conversational chatbots.Chatbot and Conversational AI
Gradio can be integrated with tools like Vertex AI Conversation to build chatbots that can answer user questions using enterprise data. This integration allows for contextual and personalized interactions with end-users. In summary, Gradio is a powerful tool that bridges the gap between complex machine learning models and non-technical users by providing an easy-to-use framework for building interactive web interfaces. Its versatility, ease of use, and strong integration capabilities make it a favorite among Python developers and machine learning practitioners.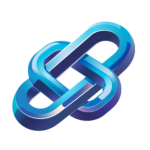
Gradio - User Interface and Experience
Gradio Overview
Gradio is an open-source Python library that simplifies the creation of user-friendly web interfaces for machine learning models, APIs, and any Python functions. Here’s a detailed look at its user interface, ease of use, and overall user experience:User Interface
Gradio’s user interface is designed to be intuitive and engaging. It offers several core classes and components that make building interactive applications straightforward:Interface Class
This high-level abstraction allows developers to quickly create demos for any Python function by specifying the input and output components. It handles the layout automatically, making it ideal for simple, single-function applications.Blocks Class
For more complex applications, the Blocks class provides a lower-level API, offering greater flexibility and control. Developers can arrange multiple components, group them in custom layouts, and define interactions between them.ChatInterface Class
This is a specialized class for conversational applications, simplifying the creation of chatbots and conversational agents by managing chat history and responses automatically.Components and Interactivity
Gradio includes a variety of interactive components that enhance the user experience:Textbox
Allows users to input text data, supporting single-line and multi-line inputs, and can be customized with placeholders, labels, and default values.Buttons
Triggers specific actions or functions when clicked, commonly used for initiating processes like data submission or model inference.Sliders
Enables users to input numerical values within a specified range, adding an interactive element to the interface.Image, Audio, and Video Inputs
Supports tasks such as image editing, audio classification, and video processing, making it versatile for various machine learning applications.Output Options
Displays outputs in formats like text, images, and interactive charts, helping users easily understand model predictions.Ease of Use
Gradio is known for its ease of use, particularly in the context of machine learning model deployment:Rapid Setup
Developers can set up an interactive app with just a few lines of code. Gradio allows you to define your model and specify the input and output components, generating the interface instantly.Minimal Code
The library requires minimal setup, making it easy to build fully functional apps quickly. For example, you can create a basic greeting app or a text generation model interface with only a few lines of code.User Experience
The user experience with Gradio is enhanced by several features:Modern Design
Gradio 5 introduces modernized component designs and built-in themes, which enhance the visual appeal and usability of applications.Performance Improvements
Server-side rendering (SSR) in Gradio 5 ensures applications load almost instantaneously in the browser, eliminating loading delays.Low-Latency Streaming
Support for low-latency streaming allows for real-time applications such as webcam-based object detection, video streaming, and conversational chatbots.Customization and Styling
Developers can customize the interface using custom CSS and Markdown features, ensuring both utility and a pleasing aesthetic. Overall, Gradio provides a seamless and interactive way to showcase machine learning models and other Python functions, making it an invaluable tool for developers who need to create user-friendly web interfaces quickly and efficiently.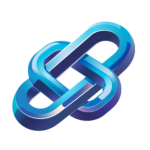
Gradio - Key Features and Functionality
Gradio Overview
Gradio is an open-source Python library that simplifies the creation of user-friendly web interfaces for machine learning models, APIs, and data applications. Here are the main features and how they work:Core Classes and Interfaces
Gradio provides several core classes that make building interactive applications straightforward:Interface
This class allows you to wrap any Python function with a UI. You can pass the function, input components, and output components to create a simple and intuitive interface. For example, you can create a text-based greeting app with just a few lines of code. “`python import gradio as gr def greet(name): return “Hello ” name “!” demo = gr.Interface(fn=greet, inputs=”text”, outputs=”text”) demo.launch() “`Blocks
This class offers more flexibility and control over the layout and interactions within the application. It supports complex interactions, such as updating properties or visibility of components based on user actions. This is useful for building more customized and complex applications.Interactive Components
Gradio includes a variety of interactive components that help in creating intuitive user experiences:Textbox
Allows users to input text data, either as a single line or multi-line input. It can be customized with placeholders, labels, and default values. “`python import gradio as gr def greet(name): return f”Hello, {name}!” with gr.Blocks() as demo: name_input = gr.Textbox(label=”Enter your name”) greeting_output = gr.Textbox(label=”Greeting”) greet_button = gr.Button(“Greet”) greet_button.click(greet, inputs=name_input, outputs=greeting_output) demo.launch() “`Button
Triggers specific actions or functions when clicked. It is commonly used for initiating processes like data submission or machine learning model inference. “`python import gradio as gr def process_data(): return “Data processed successfully!” with gr.Blocks() as demo: process_button = gr.Button(“Process Data”) result_output = gr.Textbox(label=”Result”) process_button.click(process_data, outputs=result_output) demo.launch() “`Slider
Enables users to select a numerical value within a specified range. This is useful for adjusting parameters like thresholds or learning rates. “`python import gradio as gr def adjust_value(value): return f”Selected value: {value}” with gr.Blocks() as demo: value_slider = gr.Slider(minimum=0, maximum=100, step=1, label=”Adjust Value”) value_output = gr.Textbox(label=”Output”) value_slider.change(adjust_value, inputs=value_slider, outputs=value_output) demo.launch() “`Dropdown
Presents a list of options from which users can select. It is ideal for scenarios where a predefined set of choices is available. “`python import gradio as gr def select_option(option): return f”You selected: {option}” with gr.Blocks() as demo: options = [“Option 1”, “Option 2”, “Option 3″] option_dropdown = gr.Dropdown(choices=options, label=”Select an Option”) selection_output = gr.Textbox(label=”Selection”) option_dropdown.change(select_option, inputs=option_dropdown, outputs=selection_output) demo.launch() “`Checkbox
Allows users to make binary choices, such as enabling or disabling a feature. “`python import gradio as gr def toggle_feature(is_enabled): return “Feature enabled” if is_enabled else “Feature disabled” with gr.Blocks() as demo: feature_checkbox = gr.Checkbox(label=”Enable Feature”) status_output = gr.Textbox(label=”Status”) feature_checkbox.change(toggle_feature, inputs=feature_checkbox, outputs=status_output) demo.launch() “`Integration with AI Models and APIs
Gradio makes it easy to integrate AI models and APIs into your applications:Generative AI Agents
You can integrate Gradio with generative AI agents, such as those built on Vertex AI Conversation, to create chatbots that can answer user questions using various data sources. This integration allows for contextual and personalized interactions with end-users.OpenAI API
The `openai-gradio` package enables developers to create machine learning apps powered by OpenAI’s API. This includes support for voice chat capabilities using real-time models.Gradio API (Python Client)
The Gradio API allows for programmatic interaction with Gradio applications, enabling remote communication, automation, and integration with other systems:Remote Interactions
You can connect to and control Gradio applications from anywhere, making it useful for deploying machine learning models as remote services.Automation and Testing
The API allows you to send inputs to the Gradio app, retrieve outputs, and automate testing workflows. This is particularly useful for automating tasks and ensuring the application works as expected.Data Integration
The API facilitates connecting Gradio apps with other systems, such as data preprocessing or external databases, making it part of a larger pipeline.Deployment and Sharing
Gradio applications can be easily deployed and shared:Local Deployment
You can launch a Gradio app locally and share it via a public link, allowing others to interact with the model remotely.Hugging Face Spaces
Gradio interfaces can be permanently hosted on Hugging Face Spaces, providing a link that can be shared with others. This allows for seamless sharing and collaboration. In summary, Gradio offers a powerful set of tools for building interactive web interfaces for machine learning models and data applications, with a focus on ease of use, flexibility, and integration with various AI models and APIs.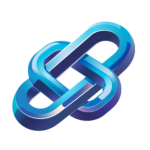
Gradio - Performance and Accuracy
Performance of Gradio 5
Gradio 5 has made significant strides in improving the performance of machine learning web applications. Here are some key enhancements:Server-Side Rendering (SSR)
Server-Side Rendering (SSR): Gradio 5 incorporates SSR, which allows applications to load almost instantaneously in the browser, eliminating previous loading delays.Low-Latency Streaming
Low-Latency Streaming: The new version supports low-latency streaming, enabling real-time applications such as webcam-based object detection, video streaming, and conversational chatbots. This is achieved through the use of base64 encoding, websockets, and support for WebRTC via custom components.Accuracy and Reliability
In terms of accuracy and reliability, Gradio 5 has addressed several critical areas:Security Improvements
Security Improvements: A comprehensive security audit by Trail of Bits identified and resolved potential vulnerabilities, ensuring safer deployment of applications. Fixes include resolving issues like the `Dropdown` component pre-process step not limiting values to those in the dropdown list, which is now fixed in Gradio 5.0.Modernized Component Design
Modernized Component Design: Core components such as Buttons, Tabs, and Sliders have been refreshed with a modern design, enhancing the visual appeal and usability of applications. Additionally, new built-in themes make it easier to create fresh-looking apps without extensive customization.Limitations and Areas for Improvement
Despite the improvements, there are some limitations and areas where Gradio 5 could be enhanced:Scalability for Large-Scale Applications
Scalability for Large-Scale Applications: While Gradio 5 is production-ready, it may not be adequate for building apps that need to interface with hundreds of thousands or millions of users. For such large-scale applications, additional expertise in frontend development might be necessary.Flexibility in UI Features
Flexibility in UI Features: Gradio can be limiting if users demand more sophisticated UI features. It is better suited for proof-of-concept (POC) type apps or basic interactivity rather than highly customized UIs.Future Enhancements
Future Enhancements: Gradio 5 is set to receive further updates, including support for multi-page apps, native navbars and sidebars, more media components, and improved DataFrame components. These upcoming features aim to address some of the current limitations and expand the capabilities of Gradio.Additional Features
Gradio 5 also introduces several innovative features that enhance its usability and functionality:AI Playground
AI Playground: This feature allows developers to generate or modify Gradio applications using natural language prompts, facilitating rapid prototyping and experimentation. Developers can preview the app right in their browser immediately.Integration and Automation
Integration and Automation: Gradio can function as both a user-facing application and a backend service, supporting programmatic interaction through its API. This makes it useful for remote API calls and automated workflows. Overall, Gradio 5 offers significant improvements in performance, security, and user experience, making it a powerful tool for building interactive machine learning web applications, although it still has some limitations, particularly in scalability and UI flexibility for very large or highly customized applications.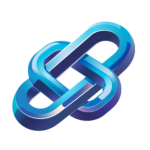
Gradio - Pricing and Plans
Pricing Structure of Gradio
Custom Pricing
Gradio does not offer predefined pricing plans or tiers. Instead, it provides custom pricing for its software, which means you need to get a quotation based on your specific requirements.No Free Plan
Gradio does not offer a free plan. If you are interested in using Gradio, you will need to contact them for a custom quote.No Free Trial
There is no free trial available for Gradio, so you cannot test the service without committing to a purchase.Hosting and Deployment Options
While Gradio itself does not have different pricing tiers, you can deploy Gradio applications through other services. For example, you can host your Gradio interfaces on platforms like Hugging Face Spaces or use managed services like Elestio, which offer predictable pricing that includes compute, storage, bandwidth, updates, security, and maintenance. However, these costs are associated with the hosting service rather than Gradio directly.Summary
In summary, Gradio’s pricing is quotation-based and does not include free plans or trials. For more detailed pricing information, you would need to contact Gradio directly.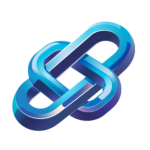
Gradio - Integration and Compatibility
Gradio Overview
Gradio, an open-source Python library, is highly versatile and integrates well with a variety of tools and platforms, making it a powerful tool for building interactive AI applications.
Integration with Other Tools
Gradio can be integrated with several other tools and services to enhance its functionality:
- Large Language Models (LLMs): Using the `gradio-tools` library, Gradio apps can be converted into tools that can be leveraged by LLM-based agents. This allows for tasks such as transcribing voice recordings, applying OCR to documents, and more.
- Weights & Biases (W&B): Gradio demos can be easily integrated into W&B dashboards. This is achieved by calling the `integrate` method with the W&B API, allowing for the creation of demos within W&B reports or dashboards. Additionally, W&B plots can be embedded within Gradio apps using HTML blocks.
- Hugging Face Spaces: Gradio apps can be hosted on Hugging Face Spaces, which provides a free hosting option. These apps can be embedded directly into blogs, websites, or documentation using specific HTML tags.
- Multi-Provider Support: The `ai-gradio` library, built on top of Gradio, supports seamless integration with over 15 AI providers, including OpenAI GPT models, Google Gemini, Anthropic Claude, and more. This allows for multi-modal interactions such as text, voice, image, and video inputs.
Compatibility Across Platforms and Devices
Gradio ensures broad compatibility across different platforms and devices:
- Python Compatibility: Gradio requires Python 3.10 or later to function, ensuring it works with the latest Python versions.
- Cross-Platform Deployment: Gradio apps can be deployed across various platforms, including web, desktop, and mobile devices. The `ai-gradio` library, for instance, supports cross-platform deployment and includes features like WebRTC voice chat support.
- Node.js and npm: For using custom components, Gradio requires Node.js v18 , npm 9 , and Gradio 4.0 (preferably Gradio 5.0 ). This ensures compatibility with the latest frontend development tools.
- Browser Automation: The `ai-gradio` library also supports browser automation, which can be useful for automating interactions within web-based Gradio applications.
Additional Features
Gradio’s API plays a crucial role in its integration and compatibility. The Gradio API allows for remote interactions, automation, and data integration, making Gradio applications versatile and extensible. This API enables developers to connect to and control Gradio applications programmatically, which is useful for automation, testing, and integrating with other systems.
Conclusion
In summary, Gradio’s ability to integrate with various tools and its compatibility across different platforms and devices make it a highly effective and flexible library for building interactive AI applications.
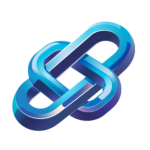
Gradio - Customer Support and Resources
Customer Support Options
Gradio, a platform for building interactive machine learning and AI applications, offers several customer support options and additional resources to help developers effectively use their tools.Community Support
Gradio has an active community that can be a valuable resource for developers. For general questions or help, users can join the Gradio Discord server, where the community and the Gradio team are available to assist.Documentation and Guides
Gradio provides extensive documentation and guides that cover various aspects of building and deploying applications. These include tutorials on creating chatbots, building web applications, and using the Gradio Python client. For example, the guide on creating a chatbot fast outlines how to use `gr.ChatInterface` and integrate additional features like returning files or Gradio components.Tutorials and Examples
The Gradio website and associated resources offer numerous tutorials and examples to help developers get started. These tutorials cover topics such as building the first web application, using the Gradio Python client, and configuring the development environment. For instance, the “Getting Started with the Gradio Python client” guide shows how to use any Gradio app as an API.API and Client Resources
For developers looking to integrate Gradio applications via APIs, the Gradio Python client is a key resource. This client allows users to interact with Gradio apps programmatically, whether they are hosted on Hugging Face Spaces or other servers. Detailed examples are provided to demonstrate how to use this client effectively.Version Updates and Release Notes
Gradio keeps users informed about new features and updates through their documentation and blog posts. For example, the guide on “Introduction to Gradio for Building Interactive Applications” covers the latest enhancements in Gradio 5, including performance improvements, enhanced security measures, and AI-powered app creation.Code Repositories and GitHub
Developers can also find support by exploring Gradio’s GitHub repository, where they can access the source code, report issues, and see the latest changes and updates. This is particularly useful for those who want to contribute to the project or need to troubleshoot specific issues.Conclusion
By leveraging these resources, developers can effectively engage with Gradio’s tools, resolve issues, and build interactive and powerful AI-driven applications.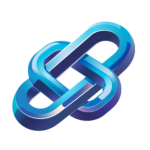
Gradio - Pros and Cons
Advantages
Ease of Use and Quick Setup
Gradio is known for its simplicity and ease of use, allowing developers to create interactive UIs for machine learning models with minimal code. It requires only a few lines of Python code to set up an interface, making it particularly friendly for data scientists and those with limited web development experience.
Interactive Components
Gradio supports a variety of input types such as text, images, audio, video, and files, which is ideal for various ML applications including NLP, computer vision, and audio processing. It also offers output options like text, images, and interactive charts, making it easy for users to interact with and understand model predictions.
Blocks API
The Blocks API in Gradio enables the creation of multistep workflows, complex layouts, and multipage applications. This feature allows for precise control over UI components and event-driven functions, which can handle complex input-output combinations.
Security Features
Gradio includes security features like password protection and encryption, ensuring a secure environment for application deployment. This is particularly important for protecting sensitive data and models.
Integration with Hugging Face
Gradio has a tight integration with Hugging Face’s model hub, allowing developers to incorporate AI models into their applications with minimal effort. This integration reduces development time and simplifies the process for those building on Hugging Face’s ecosystem.
Community and Corporate Use
Gradio is used by both corporate entities and academic institutions, such as MIT and Stanford, which adds to its credibility and reliability. It is also useful for identifying biases and testing models against adversarial attacks.
Disadvantages
Limited Advanced Customization
One of the main drawbacks of Gradio is its limited advanced customization features. While it is great for simple, interactive UIs, it may not be as flexible as other frameworks like Streamlit when it comes to complex customization needs.
Code Management
As applications grow in complexity, managing the code, especially with the Blocks API, can become challenging. Developers need to adopt best practices for code organization to handle larger projects effectively.
Smaller Community and Integrations
Compared to Streamlit, Gradio has a smaller community and fewer integrations. This can be a disadvantage for developers who rely on extensive community support and a wide range of integrations for their projects.
Conclusion
In summary, Gradio is an excellent choice for developers who need to quickly create interactive UIs for machine learning models with a focus on ease of use, security, and integration with Hugging Face. However, it may not be the best option for projects that require advanced customization or a larger community support network.
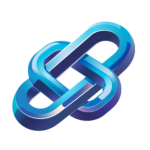
Gradio - Comparison with Competitors
When Comparing Gradio to Other AI-Driven Developer Tools
When comparing Gradio to other AI-driven developer tools in the category of building and deploying machine learning models, several key features and alternatives stand out.
Unique Features of Gradio
- Fast and Easy Setup: Gradio is known for its simplicity, allowing users to set up a web interface for their machine learning models with just a few lines of code. It can be installed using
pip install gradio
and integrated seamlessly with any Python library. - Public Sharing and Hosting: Gradio enables users to generate a shareable public link for their interfaces and offers permanent hosting options on Hugging Face Spaces.
- Embedded Interfaces: Gradio interfaces can be embedded in Python notebooks or presented as standalone webpages, making it versatile for different use cases.
- Integration with Hugging Face: Gradio’s tight integration with Hugging Face’s model hub allows developers to incorporate AI models into their applications with minimal effort, reducing development time significantly.
Alternatives and Competitors
Streamlit
- Streamlit is another popular tool for transforming data scripts into interactive web applications. It is particularly useful for machine learning model deployment and data visualization. Unlike Gradio, Streamlit requires a bit more setup but offers powerful features for building interactive applications. Streamlit was acquired by Snowflake in 2022, which has further enhanced its capabilities.
- Key Difference: Streamlit focuses more on data visualization and interactive applications, whereas Gradio is more geared towards quick and easy deployment of machine learning models.
AI Squared
- AI Squared specializes in integrating AI into business applications across various sectors. It provides technologies that enable the embedding of AI insights into front-end applications, facilitating a collaborative environment for AI consumers and developers. AI Squared is more focused on decision-making and workflow optimization through AI adoption.
- Key Difference: AI Squared is more business-oriented and focused on integrating AI into existing workflows, whereas Gradio is more developer-centric and focused on demonstrating and deploying machine learning models.
Other Considerations
No-Code/Low-Code Development
- Gradio 5 introduces an “AI Playground” that allows users to create and preview AI-powered applications using natural language prompts, similar to other no-code or low-code development tools. This feature makes Gradio even more accessible to a broader audience.
Open-Source and Free
- Gradio is an open-source tool, available for free, which makes it highly accessible to individual developers, educational institutions, and research organizations. This is a significant advantage over some other tools that may require subscription fees.
Conclusion
In summary, Gradio stands out for its ease of use, seamless integration with Python libraries, and public sharing capabilities. While Streamlit offers more advanced features for interactive applications and data visualization, AI Squared is more suited for business applications and workflow optimization. Gradio’s unique features, especially its integration with Hugging Face and the new AI Playground, make it a compelling choice for machine learning practitioners looking to quickly deploy and demonstrate their models.
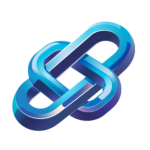
Gradio - Frequently Asked Questions
Here are some frequently asked questions about Gradio, along with detailed responses to each:
What is Gradio and what is it used for?
Gradio is a tool designed to simplify the process of building user interfaces (UIs) for machine learning models. It allows developers to create interactive and user-friendly web applications for their ML models quickly and easily, supporting various input types such as text, images, audio, video, and files.Does Gradio offer a free plan or trial?
No, Gradio does not offer a free plan or a free trial. The pricing for Gradio is custom and quotation-based, meaning you need to contact the vendor for specific pricing details.What are the key features of Gradio?
Gradio supports a range of interactive components, including text, image, audio, video, and file inputs. It also offers various output options like text, images, and interactive charts. Additional features include preprocessing and postprocessing, styling demos, queuing users, iterative outputs, and progress bars. Gradio also integrates well with Hugging Face’s model hub, making it easy to incorporate AI models into applications.What are the system requirements for using Gradio?
To use Gradio, especially for custom components, you need to have Python 3.10 , Node.js v18 , and npm 9 . For the latest features, Gradio 5.0 is recommended. These requirements ensure compatibility and smooth functionality of the custom components.How do I create custom components in Gradio?
Creating custom components in Gradio involves several steps. You need to ensure you have the necessary dependencies installed, such as Python 3.10 and Node.js v18 . You can start by cloning existing custom component repositories or using built-in templates. You must implement methods like `preprocess`, `postprocess`, `example_payload`, and `example_value`. For components without a data model, you need to define `api_info`, `flag`, and `read_from_flag` methods. You can also use the `gradio cc dev` command to run a development server for your custom component.What is the AI Playground in Gradio 5?
The AI Playground in Gradio 5 is an experimental feature that allows users to create and preview AI-powered applications using natural language prompts. This feature is similar to no-code or low-code development tools but offers web-based previews that can run directly in the browser, enabling the creation of production-ready ML web applications with minimal effort.How does Gradio integrate with Hugging Face’s model hub?
Gradio’s integration with Hugging Face’s model hub is a key advantage. Once an AI model is uploaded to the Hugging Face repository, developers can incorporate it into their Gradio applications with just a few commands or even spoken instructions. This integration significantly reduces development time and simplifies the process of building ML applications within the Hugging Face ecosystem.What kind of support does Gradio offer?
Gradio provides online support through a ticket system. This means you can submit tickets for any issues or questions you have, and the support team will address them accordingly.Can I deploy Gradio applications in the cloud?
Yes, Gradio applications are cloud-hosted, making it easy to deploy and manage your ML web applications in a scalable and secure environment.How do I share my custom Gradio components with the community?
To share your custom Gradio components, it is recommended to publish your package to PyPi and host a demo on Hugging Face. This allows anyone to install or try out your component easily.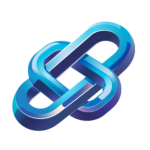
Gradio - Conclusion and Recommendation
Final Assessment of Gradio
Gradio is an exceptional tool in the Developer Tools AI-driven product category, particularly for machine learning developers and enthusiasts. Here’s a comprehensive overview of its benefits and who would most benefit from using it.Ease of Use and Setup
Gradio stands out for its simplicity and ease of setup. You can install it using pip with just a few lines of code, making it accessible even to those who are not deeply familiar with web development.Compatibility and Flexibility
Gradio works seamlessly with any Python library, allowing developers to integrate it into their existing projects without hassle. It also supports embedded interfaces within Python notebooks or as standalone webpages, providing flexibility in how the models are presented.Public Sharing and Hosting
One of the key features of Gradio is its ability to generate a shareable public link for remote access, facilitating collaboration and public demonstrations of machine learning models. Additionally, users can opt for permanent hosting on Hugging Face Spaces, ensuring their interfaces are always available.Benefits for Developers
Gradio simplifies the process of creating machine learning demonstrations with minimal coding, making it an ideal tool for developers who want to showcase their models quickly. It enhances accessibility by making these models understandable to a non-technical audience, which is crucial for collaboration and feedback. The tool also provides a platform to showcase work to the wider community, which can be beneficial for visibility and feedback.Gradio Lite and Advanced Features
For those looking to avoid server infrastructure, Gradio Lite is a valuable option. It runs in the browser, eliminating the need for server setup and reducing costs. This version also enhances privacy and security since all processing occurs within the user’s browser.Integration with Hugging Face
Gradio 5, the latest version, integrates tightly with Hugging Face’s model hub, allowing developers to incorporate AI models into their applications with minimal effort. This integration significantly reduces development time and simplifies the process of building production-ready machine learning web applications.Deployment Options
For deployment, Gradio can be used with various platforms like Modal, which offers a serverless architecture, rapid deployment, and cost-effective usage. This makes it easy to get demos online quickly and manage updates efficiently.Who Would Benefit Most
- Machine Learning Developers: Those who need to quickly demonstrate their models will find Gradio invaluable.
- Researchers: Researchers can use Gradio to share their models and collaborate with peers more effectively.
- Educational Institutions: Gradio’s ease of use and free availability make it a great tool for educational purposes, allowing students to explore and demonstrate machine learning concepts.
- Non-Technical Audiences: By making machine learning models accessible through a user-friendly web interface, Gradio helps non-technical stakeholders understand and interact with these models.